Creating a Payment
Create a new payment in the system.
Access Endpoint URL:https://rest.everyware.com/api/Default/CreatePayment [POST]
The CreatePayment method allows you to accept online credit card or bank account payments.
Inbound Parameters
Parameters should be passed in a single JSON-body object.
Parameter Name | Description | Optional/Required |
---|---|---|
FirstName | The first name of the customer making the payment. | Required |
LastName | The last name of the customer making the payment. | Required |
Address1 | Line one of the address of the customer making the payment. May not always be required depending on the gateway. | Optional |
Address2 | Line two of the address of the customer making the payment. | Optional |
City | The city in the address of the customer making the payment. | Optional |
StateCode | The state code in the address of the customer making the payment. Format: 2-letter code (TX, FL, etc.) |
|
PostalCode | The postal/zip code in the address of the customer making the payment. | Optional |
CountryCode | The country code in the address of the customer making the payment. Example: United States = US | Optional |
The email address of the customer making the payment. Format: [email protected] | Required | |
MobilePhone | The mobile phone number (10-digits max, no formatting) of the customer making the payment. If Everyware messaging and invoicing are not being used, a unique 10-digit number that is not a phone number may be used. Speak with your Everyware representative for more information. Example: 3055551212 | Required |
CCNumber | The credit card number of the customer making the payment. No spaces should be included in the parameter. | π³ REQUIRED FOR CREDIT CARD METHOD |
ExpirationMonth | The month in which the paying customer's credit card will expire. Months 1-9 require a preceding 0. Examples: "05", "11" | π³ REQUIRED FOR CREDIT CARD METHOD |
ExpirationYear | The year in which the paying customer's credit card will expire. Must be provided in 2-digit format. Example: 2016 = "16" | π³ REQUIRED FOR CREDIT CARD METHOD |
CVV | The verification code on the paying customer's credit card. For Visa or Master Card, this is a 3-digit number. For American Express, this is a 4-digit number. | π³ REQUIRED FOR CREDIT CARD METHOD |
CardType | The brand of the card. | Optional |
CardToken | The token for the card to be charged, as returned from the Create Payment API. | Optional |
ACHToken | The token generated from the individual's banking information. | Optional |
AccountName | The name associated with the bank account. | Optional |
AccountNumber | The bank account number of the customer. Used for ACH transactions. When testing in the sandbox, any account number between four and eleven digits may be used. | π¦ REQUIRED FOR ACH METHOD |
RoutingNumber | The bank routing number for the customer's bank account. Used for ACH transactions. When testing in the sandbox, any valid US routing number may be used. | π¦ REQUIRED FOR ACH METHOD |
AccountType | The type of bank account. | Optional |
PaymentType | Default value set to "CreditCard". If doing ACH transactions, set value to "ACH". | Optional |
Amount | The amount that is to be paid by the customer. Example: 1.00 | Required |
Description | A description of the processed order. | Optional |
OrderNumber | This should be a unique value your application defines which is associated with the invoice that appears on the top of the customer facing invoice as "Invoice #" and throughout the Everyware portal. | Optional |
ChargeType | Charge β Charge a Credit Card or ACH account in a single transaction. For ACH π¦ - Use Charge Authorize β authorize a Credit Card for a specific amount but doesn't charge it right away. Default value is Charge. Use this parameter instead of IsAuthOnly unless your Everyware Implementation Specialist indicates otherwise. | Optional |
IsEmailReceipt | True/False. If true, sends a receipt via automatic email to the email address provided above. Default value set to true. | Optional |
IsSMSReceipt | True/False. If true, sends a receipt via SMS to the mobile phone number provided above. Default value set to true. | Optional |
CreateToken | True/False. Set to true to tokenize credit card or ACH account as it is run. Set to false if tokenization is not desired. Default value set to false. | Optional |
StatementDescriptor | In payment receipts and receipt text messages, the statement descriptor is what will show on the customer's bank statement. Note: *Make sure this matches with what will actually show on your customer's bank statement to avoid confusion. Otherwise, set up custom descriptors for your sales site(s) with your Everyware rep. | Optional |
IndividualID | The ID associated with the customer making the payment in the Everyware system. | Optional |
ExternalID | A unique identifier from an external system associated with the payment that Everyware can store. | Optional |
IsAuthOnly | True/False Determines whether this is simply authorization and not capture. Used only for specific gateways. Default value is set to false. | Optional |
ConvAmount | The dollar amount for an optional convenience fee. The settings for this fee can be adjusted in Portal. If left blank or at $0.00, it will not appear as a line item. | Optional |
TipAmount | The dollar amount that will be left as a tip. | Optional |
TaxAmount | The dollar amount for the taxes to be charged. Settings for taxes can be adjusted in Portal. If left blank or at $0.00, it will not appear as a line item. | Optional |
SubtotalAmount | The total dollar amount for all line items excluding taxes and fees. | Optional |
DiscountAmount | The amount that will be discounted from the total charge. | Optional |
DeliveryFee | The dollar amount for the optional "Delivery Fee" line item, which can be activated and adjusted in Portal. If left blank or at $0.00, it will not appear as a line item. | Optional |
CustomFee1 | The dollar amount for an optional, custom line item. This fee type can be activated, labeled, and adjusted in the Everyware Portal. If left blank or at $0.00, it will not appear as a line item. | Optional |
CustomFee2 | The dollar amount for an optional, custom line item. This fee type can be activated, labeled, and adjusted in the Everyware Portal. If left blank or at $0.00, it will not appear as a line item. | Optional |
CustomerExternalID | A unique identifier from an external system associated with the customer making the payment that Everyware can store. | Optional |
InvoiceExternalID | A unique identifier from an external system associated with the invoice the customer is paying that Everyware can store. | Optional |
OtherAmount1 | A field that can store any other amount associated with the payment that is desired. | Optional |
OtherAmount2 | A field that can store any other amount associated with the payment that is desired. | Optional |
OtherAmount3 | A field that can store any other amount associated with the payment that is desired. | Optional |
OtherAmount4 | A field that can store any other amount associated with the payment that is desired. | Optional |
AmountPaymentFee | The fee associated with special charges for certain payment methods. If you charge 1.00 to use a card, for example, this is where you would indicate the 1.00. | Optional |
OtherAmount1Name | The name for the amount in OtherAmount1. | Optional |
OtherAmount2Name | The name for the amount in OtherAmount2. | Optional |
OtherAmount3Name | The name for the amount in OtherAmount3. | Optional |
OtherAmount4Name | The name for the amount in OtherAmount4. | Optional |
NamePaymentFee | The name given to the amount in the AmountPaymentFee parameter. | Optional |
Sample Use Case 1: Create Card Payment
Use a customer's card as the payment method.
{
"FirstName": "Suzie",
"LastName": "Sick",
"Address1": "123 Testing",
"Address2": "",
"City": "Austin",
"StateCode": "TX",
"PostalCode": "78701",
"CountryCode": "US",
"Email": "[email protected]",
"MobilePhone":"5125555555",
"CCNumber": "111111111111111",
"ExpirationMonth": "11",
"ExpirationYear": "29",
"CVV": "111",
"Amount": "1",
"Description": "New Patient Enrollment Fee",
"OrderNumber": "111111",
"ChargeType": "Charge",
"IsEmailReceipt": false,
"IsSMSReceipt": true,
"StatementDescriptor": "HelloSunshine",
"CreateToken": true
}
curl --location 'https://rest.everyware.com/api/Default/CreatePayment' \
--header 'Authorization: Basic [xxx]' \
--header 'Content-Type: application/json' \
--data-raw ' {
"FirstName": "Suzie",
"LastName": "Sick",
"Address1": "123 Testing",
"Address2": "",
"City": "Austin",
"StateCode": "TX",
"PostalCode": "78701",
"CountryCode": "US",
"Email": "[email protected]",
"MobilePhone":"5125555555",
"CCNumber": "111111111111111",
"ExpirationMonth": "11",
"ExpirationYear": "29",
"CVV": "111",
"Amount": "1",
"Description": "New Patient Enrollment Fee",
"OrderNumber": "111111",
"ChargeType": "Charge",
"IsEmailReceipt": false,
"IsSMSReceipt": true,
"StatementDescriptor": "HelloSunshine",
"CreateToken": true
}
{
"IsSuccess": true,
"Message": "The credit card has been accepted.",
"Data": {
"TransactionStatus": true,
"InvoiceNumber": "ew_3JN25fAawEMtODxF3OEtn7Q1",
"InvoiceID": "ew_3JN25fAawEMtODxF3OEtn7Q1",
"TokenStatus": true,
"TokenResult": "card_ew12345678abc"
},
"OrderNumber": "111111"
}
Sample Use Case 2: Create Bank Account Payment
Use a customer's bank account as the payment method.
{
"FirstName": "Suzie",
"LastName": "Sick",
"Address1": "123 Suzie St",
"Address2": "",
"City": "Boca",
"StateCode": "FL",
"PostalCode": "33472",
"CountryCode": "US",
"PaymentType": "ACH",
"Email": "[email protected]",
"MobilePhone": "5129474078",
"AccountNumber": "1234536",
"RoutingNumber": "031176110",
"Amount": "1",
"Description": "Name of Item Purchased",
"ChargeType": "charge",
"IsEmailReceipt": false,
"IsSMSReceipt": true,
"StatementDescriptor": "HelloSunshineMaster",
"CreateToken": false
}
curl --location 'https://rest.everyware.com/api/Default/CreatePayment' \
--header 'Authorization: Basic [xxx]' \
--header 'Content-Type: application/json' \
--data-raw ' {
"FirstName": "Suzie",
"LastName": "Sick",
"Address1": "123 Suzie St",
"Address2": "",
"City": "Boca",
"StateCode": "FL",
"PostalCode": "33472",
"CountryCode": "US",
"Email": "[email protected]",
"MobilePhone": "5129474078",
"AccountNumber": "1234536",
"RoutingNumber": "031176110",
"Amount": "1",
"Description": "Name of Item Purchased",
"ChargeType": "charge",
"IsEmailReceipt": false,
"IsSMSReceipt": true,
"StatementDescriptor": "HelloSunshineMaster",
"CreateToken": false
}
{
"IsSuccess": true,
"Message": "\r\nThe credit card has been accepted.",
"Data": {
"TransactionStatus": true,
"InvoiceNumber": "EWTest_141150e7-1cae-4236-be7a-6147098614fa",
"InvoiceID": "EWTest_141150e7-1cae-4236-be7a-6147098614fa",
"ReceiptNumber": "EWTest_141150e7-1cae-4236-be7a-6147098614fa",
"TokenStatus": false,
"TokenResult": null
},
"OrderNumber": "E366849224",
"SMSID": null
}
Example Integrated Payment Webform
Integrated payment webform using the CreatePayment API to process online card payments.
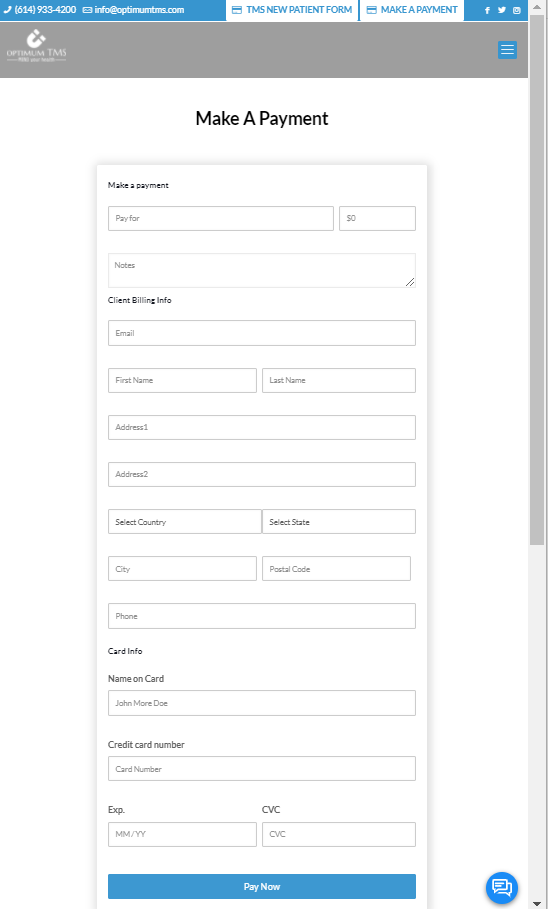
Updated 4 months ago